Week 14 coding authentication in our groups application
Share
Welcome to the week 14 thoughts and documentation. I am in the last five weeks of the program, and I’m very excited to be training most of my time to apply for roles as a software engineer in a remote situation. This week we had lectures on React Hooks, authenticating our application from a web library created by Galvanize, MongoDB, and learning more about Jason Web Tokens and algorithms.
Our group project for this week was focused on building out our login and logout, and create an account authentication, and also being able to successfully make an API call to our 3rd party API application with Open Weather. The following is the text from my journal of the work's last three group working days, including challenges and focuses for our project.
5/16/23
Today, I worked on the following:
- Getting our Docker Containers running and our Authentication
I worked with Jeanette, Liland, and Sabrina as navigators for Jason, who was the driver for today. We built out our docker.compose.yml file to be able to run our FastAPI server, Mongo, Mongo Express, and React development servers. After getting our development servers to run, we built out the backend server portion for authenticating our users. We now have a queries and routers folder in our monolith (whatevr) directory that allows us to create, get, and list user accounts. Once implemented, our application now can:
- Allow users to log in and log out.
- Require a valid token for access to specific endpoints.
- Get the current account data for a logged-in user.
- Let users sign up for new accounts.
We learned that JWTdown for FastAPI provides two routes for logging in and out: The POST /token to log in using form data sets a cookie and returns a JSON token for you to use in your API calls. DELETE /token to log out, which deletes the cookie set with logging in. We can use the authenticator when requiring a valid token to access specific endpoints.get_current_account_data method as an injected dependency. This method will return the current account data for a logged-in user or None if the user is not logged in. We used the authenticator to get the current account data for a logged-in user.try_get_current_account_data method. This method will return the current account data for a logged-in user or None if the user is not logged in. Finally, we learned we can use the authenticator to let users sign up for new accounts. Login method. This method inputs an AccountForm object containing the new account's username and password. The method will return a Token object if the login is successful or raise an exception if the login is unsuccessful. We are excited to implement Authentication in my application using JWTdown for FastAPI, making our application more secure and user-friendly.
We plan on analyzing the code Violet provided us yesterday to further build our authentication section to the backend of our application and, later this week, integrate React hooks and frontend authentication.
5/17/23
Today, I worked on the following:
- Updating our authentication functionality in our FastAPI and making sure users are authenticated, updated models, and worked on creating the API Open Weather fetch
Our group participated as a whole in updating the Authentication in our application and focusing on the query and router directory for our accounts(users), and starting the process of writing our API call for Open Weather API. Today I was the driver, writing most of the code and sharing my screen with the team. Everyone else was the navigator and provided guidance and insight for every line of code. We added a field to confirm passwords upon the creation of accounts. From there, we retested our login and logout and created account functionality. Afterward, we received consulting help from Violet on the structure of our files and directory in our project. After this, we spent the last 2 hours of our group work figuring out how to connect our database (MongoDB) to our Python environment. We want to return the weather for all our users based on the zip code they enter upon account creation.
We attempted numerous different codes in our weather.py file in our router directory in hopes that it would return the correct API call using the zip code that was created for each user in our database. We are left to return the fields created in our model, but, unfortunately, we are still not returning data from our database, which is blocking our ability to perform a correct API call from open weather's API. Last, I installed the pymongo library on a new and separate branch in hopes that this would help with creating a MongoClient object and then have the ability to connect to our MongoDB database. Unforently after an hour of attempting and numerous versions of playing the pymongo in my requirements.txt, I still needed help to import the pymongo library and have it recognized in my code. I will update the team on this attempt and spend the following working day focused on connecting our MongoDB database to our Python environment to use it in our FastAPI and, additionally, spending time working on React Hooks.
5/18/23
Today, I worked on the following:
- Creating our MongoDB Compass Database, successfully fetching our API from Open Weather.
Jason was the driver today, and the rest of our group were the navigators. We, as a team, worked together on getting our accounts (users) to display in the MongoDB collection displayed on the MongoDB Compass (graphical user interface (GUI) for MongoDB that allows you to explore, query, and analyze your data). After getting this to work, we discussed a team and decided to focus on getting our API call to Open Weather to work on FastAPI. After seeing the data being returned in our API call, we successfully extracted the city, temperature (in Fahrenheit), description of the weather, time, and logo icon of the weather. In addition, we could return a five-day forecast of the weather data for a specific city. We still need to adjust the format for how the time is being produced and will do this later on the front-end side. From here, we discussed again and decided on working and building our models for the closet, clothes, and bins. We were still determining how to include some fields when using MongoDB, but after trial/error and research, we got our Bin model and created a bin to register an object id in our MongoDB compass. Our team has a three-day work break, and we have agreed to focus on Monday to progress our models further and get/post functionality to the three collections we need for our project: clothes, bins, and closets and our work day ended with the team feeling optimistic about our success in returning the correct data required from Open Weather. Our blockage is with MongoDB, and not fully grasping how it works, but every day this week, we learn more and more.
JWT - Jason Web Tokens
I've come to better understand JSON Web Tokens (JWTs) for Authentication and authorization in web applications since introducing the topic early on in Module 2. JWTs are a concise and secure way of transmitting information between parties without using cookies or sessions. They can be signed using a secret or public/private key pair and can contain a set of claims that include information about the user's ID, email, and authorization status.
One of the most significant advantages of using JWTs is that they are stateless, meaning that the server does not need to store any information about the user's session or login status. This makes JWT-based Authentication and authorization more scalable and efficient than traditional session-based approaches.
Numerous libraries and frameworks are available for implementing JWT-based Authentication and authorization in web applications, making it a widely used and well-supported technology. If you're interested in learning more about JWTs, many resources are available online, including official documentation and online courses and tutorials.
MongoDB
MongoDB is a popular NoSQL database I use to store data in flexible, JSON-like documents. This means that fields can vary from document to document, and the data structure can be changed over time, making it a good choice for applications with rapidly changing data requirements. Additionally, MongoDB is designed to scale horizontally across multiple servers, making it a good choice for applications that handle large amounts of data and high traffic levels.
MongoDB also offers a variety of features that make it a powerful tool for data management, including dynamic querying, indexing, and aggregation. With dynamic querying, I can query the data in MongoDB using a variety of operators and expressions without having to define a specific schema or data model ahead of time. Indexing allows me to create indexes on particular fields to improve query performance, while aggregation enables me to perform complex operations on large datasets, such as grouping, sorting, and filtering.
Furthermore, MongoDB has a robust ecosystem of tools and integrations that make it easy to work with and integrate into my existing applications. These include drivers for various programming languages and integrations with popular frameworks and tools, such as Django, with which I have become proficient while working in the Hack Reactor program.
Big O and Algorithms
Big O notation is a mathematical notation used to describe the upper bound of the time complexity of an algorithm. It is commonly used in computer science to analyze the performance of algorithms in terms of their input size. In essence, Big O notation helps me understand how an algorithm will perform as the size of its input grows.
For example, if I have an algorithm that takes n steps to complete, where n represents the size of the input, its time complexity is O(n). This means that the time it takes to run the algorithm is proportional to the input size.
By analyzing the time complexity of algorithms using Big O notation, I can choose the most efficient algorithm for a given problem and optimize my code for performance. This can be especially important when dealing with large datasets or complex algorithms, where even minor performance improvements can significantly impact.
Big O notation can be used to classify algorithms based on their efficiency. The most common classes of complexity are:
- O(1) – constant time complexity
- O(log n) – logarithmic time complexity
- O(n) – linear time complexity
- O(n log n) – linearithmic time complexity
- O(n²) – quadratic time complexity
- O(2ⁿ) – exponential time complexity
Understanding Big O notation helps identify bottlenecks in code and optimize algorithms for better performance. It can help me make informed decisions about which algorithm to use for a given problem and how to optimize my code for efficiency. When analyzing various codes from this perspective, it can get very complex in the logic of time spent and all the possibilities. I write this to remind myself that practicing this logic/perspective when creating and constructing code will allow me to understand algorithms more efficiently.
For example, I learned about economics in 1975, I learned about economics and MIT's Economic Projection and Policy Analysis (EPPA) model. The EPPA model is a computable general equilibrium (CGE) model that simulates the interactions of various economic agents, including households, firms, and governments. It is used to study economic issues such as climate change, resource depletion, and inequality.
The EPPA model has been used to predict changes in the world economy. In 2014, MIT economists used the model to predict a 20% chance of societal collapse by 2040 due to climate change, resource depletion, and inequality. I learned about this prediction from a recent Economics Explained video, which led me to ponder the individuals involved in creating this model and its time complexity.
React Hooks
React Hooks are a new feature added in React 16.8 that allows developers to use state and other React features in functional components. Before the introduction of hooks, functional components were limited in their ability to handle state and lifecycle methods, making them less flexible than their class-based counterparts. With hooks, developers can use features like useState, useEffect, and useContext to manage state, perform side effects, and share data between components. This simplifies the development process and makes it easier to manage complex components. Overall, React Hooks are a powerful tool that can significantly improve the functionality and efficiency of React applications.
One of the key benefits of using hooks is that they allow developers to write more declarative and less verbose code. By using hooks, developers can reduce the amount of boilerplate code needed to manage state and lifecycle methods, resulting in code that is easier to read and maintain. Additionally, hooks can help improve the performance of React applications by reducing the number of unnecessary re-renders that occur when the state is updated.
Going into week 15
Val and myself will be greeted by Andres Ospina this week here in Santa Rosa de Cabal to work on business tasks and have the opportunity to show him a little bit of the town. Also, I have another friend visiting us on Friday for a few days who will be visiting from Medellín. This week the focus for our group project will be enhancing our backend endpoints regarding the data we extract from our API calls and successfully connecting it with database collections created in our Mongo DB. In addition, since this is a five-day week as opposed to last week's four-day week, we will have time to work on our application's front-end build using React. Looking forward, and now I'm off to work on my documentation and homework to present to the Father of our church Parroquia Santa Maria del Monte Carmelo. Ciao!
Ready to work with Xander Clemens?
I'd be happy to discuss your project and how we can work together to create unique, fun and engaging content.
Go ahead and click here to be taken to my business service page and see what I can do for you. Book a call with me now. Looking forward to chatting soon!
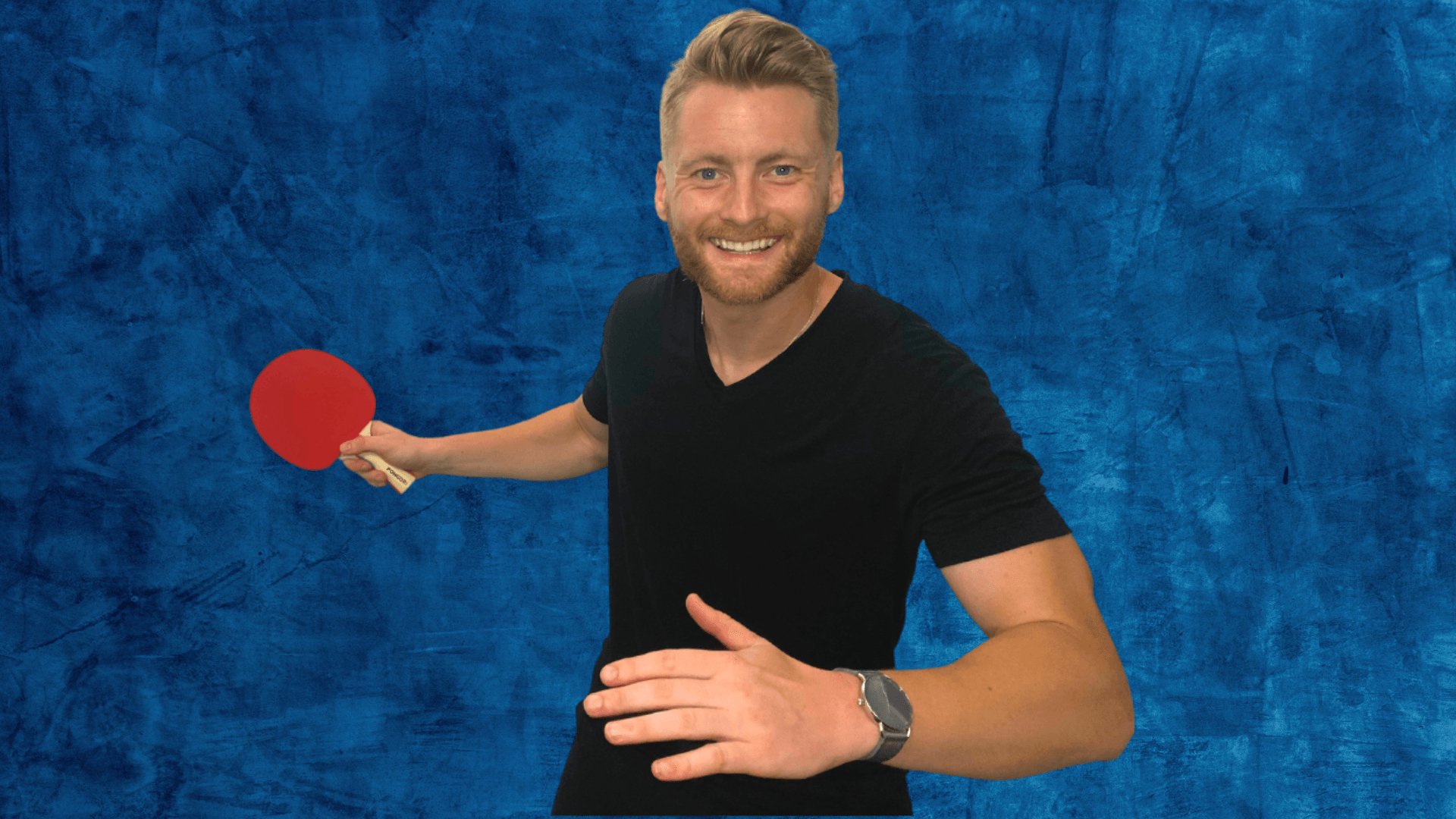