Spring Break Week in Hack Reactor
Share
This week was spring break, so a whole week off from the program as the employees for Hack Reactor/Galvanize had the same week off. While some individuals took the entire week off from being plugged into the world of using tech/coding/programming, I spent my week off getting an extra hour of sleep each day, meditating, completing LinkedIn learning courses on React, tech trends, API's and working on my side project managing work here in Colombia. The following are all the learnings this week and a section detailing what has been learned in Module 2 up until now. Let's dig into it!
Linkedin Prompt AI Generating
In the LinkedIn Learning course "Introduction to Prompt Engineering for Generative AI," I learned about using natural language processing (NLP) in generative AI. The course covered the basics of NLP and how it can be used to generate text and other content. I also learned how to use prompt engineering to fine-tune generative AI models for specific tasks. The course provided me with a comprehensive overview of the use of NLP in generative AI and its potential applications in various industries. Overall, I came away from the course with a better understanding of how AI can automate tasks and create new content without human intervention.
React.js Essential Training
In the LinkedIn Learning course "React.js Essential Training," I learned how to use React - a widely used JavaScript library - to create interactive web applications. The course is perfect for beginners or those with experience in web development, as it covers everything from the basics of React to more advanced topics such as React Router and Redux.
The course starts by introducing the core concepts of React, including components, props, states, and events. The instructor does an excellent job of breaking down each concept and providing real-world examples that help to reinforce the learning. I appreciated the course's hands-on approach, as I could follow along with the instructor and practice what I learned as I went.
As the course progresses, it covers more advanced topics such as React Router and Redux. These topics are essential to creating more complex and interactive web applications. The instructor provides clear explanations and examples, making it easy to follow even if you are new to these concepts.
One of the best things about this course is that it provides plenty of practice opportunities. The instructor includes multiple coding exercises throughout the course, allowing you to apply what you've learned and build your React applications. This hands-on learning approach helped me better understand how React works and how to use it to build real-world applications.
Overall, "React.js Essential Training" is an excellent course for anyone learning how to use React to build web applications. The instructor's clear explanations and hands-on approach make it easy to follow along, even for beginners. By the end of the course, I felt confident in my ability to build complex, interactive web applications with React, and I highly recommend this course to anyone looking to do the same.
Hands-on Introduction to React
In the "Hands-On Introduction to React" course on LinkedIn Learning, I gained valuable experience using React to create dynamic web applications. The course covered React's fundamentals, including components, props, and state, and how to create reusable UI components. In addition, I learned how to work with the React Router and Redux libraries to develop more complex applications.
The course balanced theory and practice, offering numerous coding exercises and examples to reinforce the learning. The instructor expertly broke down the core concepts of React and illustrated how they work together.
Overall, the course was incredibly helpful in learning how to use React to build modern web applications. The instructor's clear explanations and hands-on approach made it simple to follow along, even for someone with little experience in web development. I recommend this course to anyone seeking to learn how to use React.
JSX
- JavaScript Syntax Extension
- HTML & JSX
- Templating
JSX is the language that you use to write React, it stands for JavaScript Syntax Extension, but also know and JavaScript XML since it's a combination of JavaScript and something that looks like the old XHTML version of HTML.
Basically it's a way to write HTML inside JavaScript. It has a few strange rules of it's own, but it works real well.
Basic Sample
function App() {
return <h1>Meet the StarGazers</h1>;
}
export default App;
Okay, so at first, it looks like regular JavaScript, but you can see that we're using an h1 tag. This is the beauty of JSX, it lets you use both, together in almost perfect harmony.
But, there's a lot of peculiarities to the language, so let's go further.
Single Parent
<hgroup>
<h1>Meet the StarGazers</h1>
<p>Members of an <b>intergalactic alliance</b>...</p>
</hgroup>
You are only allowed to use one parent element in your HTML. So, if I were to remove the hgroup tag here, I would get an error.
Let's take a look at this in CodeSpaces. I'm going to go to the URL for the codespace.
Sometimes, people use a fragment to solve this, so instead of the hgroup tag, you would use an empty element <></>.
Self Closing Tags
return (
<hgroup>
<img src="images/group.svg" alt="StarGazer Group" />
<hgroup>
<h1>Meet the <i>{name}</i></h1>
<p>Members of an <b>intergalactic alliance</b><br />
paving the way for peace and benevolence among all species...</p>
</hgroup>
</hgroup>
);
-
<br />
or<img />
- return with
()
In JSX, all tags have to self-close. So, if I were to remove the slash in thetag, I would get an error.
The same thing would happen in an image tag.
By the way, when you want to use multi line code in JSX, just like in JavaScript, the return statement can't be on a line by itself. So you have to use parenthesis otherwise your browser's compiler will add a semicolon and break the whole thing.
Reserved Keywords
<div className="container">
<article>
...
</article>
</div>
-
className
instead ofclass
-
HTMLFor
instead offor
You can't use reserved JavaScript keywords like class or for in jsx.
Instead of class, we use className and similarly with
for, we use HTMLFor. It's something you have to remember and annoy you a bit as you write in JSX.
Expressions
const name = 'StarGazers';
...
<h1>Meet the <i>{name}</i></h1>
You can use expressions in your code, so if you create a variable for an element, you can use it in the JSX.
The expressions can be a variable name and other valid JavaScript expressions.
In React, you can reuse bits of code called components...using of two styles: classes and hooks. Let's take a look at the differences and why hooks are more dominant.
Components
- Reusable code
- Accept
props
- Return display
Before we get into classes or hooks, lets define what a component is and does.
Components are a way to create reusable code. Think of them like functions that are a bit more flexible.
Components can accept any number of inputs called props
and they return what will be displayed as part of your application.
Class Components
- Object syntax
- Still valid
- Harder to write
React has been around for quite a while and until recently, its exclusively used the object syntax to create components.
It's something that's fallen out of favor, but is still valid, but it's less familiar to modern developers.
Class Component
import React, { Component } from "react";
class Welcome extends React.Component {
constructor() {
super();
}
render() {
return <h1>Meet the StarGazers</h1>;
}
}
This is a basic class based components. Let's jump into our code.
It might look familiar if you're used to writing Class based JavaScript.
In order to use components, we import the React object and the component object from the React library.
Next we start by declaring a Class. Class names are always capitalized.
Next we use the class to extend the functionality of a React component.
We then use a special function called a constructor. The job of the constructor is to initialize the instance of the class when it is used.
Then we get into the render statement, where you output what you want React to show.
That seems like a lot for a simple component and that's the way components worked for a long time.
Passing Props
class Welcome extends React.Component {
constructor(props) {
super(props);
}
render() {
return <h1>Meet the <i>{this.props.name}</i></h1>
}
}
A component that just prints out some HTML is pretty useles, so let's take a look at how we can make it accept a parameter, like you would a function.
To do that, we can pass along props, or properties to the component, just like we would with a regular HTML tag.
In our return statement, we can use the this keyword to target the props we receive in the constructor and then display our name.
Hooks
const Welcome = (props) => {
return (
<h1>Meet the <i>{props.name}</i></h1>
)
}
Hooks allow you to use the function syntax you already know to create componets. Let's take a look at how you would use them to create this component. You'll see that it's a lot simpler.
Technically, just writing components at functions make a lot of sense, but in reality, that notation isn't what makes it a hook based component. Let's take a look at what does.
React Hooks
- Hook into React
- Access to state
- Access to lifecycle
Hooks are special functions that allow you code to hook into React's objects.
They give you access to things like state, which is how we store data in React. We'll look at that in a minute when we learn about the useState hook.
They also give you access to lifecycle methods, which are methods called when certain events happen in your application.
The second most popular hook in react is the useEffect hook. Let's take a look at what it does and why you need it.
useEffect Hook
- Side effects
- Outside scope
- Replaces lifecycles
- After render
The useEffect hook is the second most popular hook in React.
It allows you manage side effects. I'm not a fan of the term "effect" here, but it makes sense if you think of it like this.
A side effect is anythihg that deals with something outside of the scope of the current component. Like when you take a medicine and sometimes they can have side effects. Things the medicine didn't intend to do to you.
The way these used to be managed in old React was with different lifecycles methods. So you had a method called something like componentDidMount for when the component mounted and a method called componentDidUpdate for when the component updated.
Basically think it as letting you do something after rendering. React guarantees that the DOM has been updated.
But that's enough talk. Let's jump into codespaces and try out this hook.
Most of your projects will use the useEffect hook and the useStateHook together to help manage state. We'll take a look at how you can use them together in the next video.
Alright, so now that we know how React works, let's take a look at how you can work with projects.
Adding React to a Site
React has been around for so long that there's a lot of ways to work with it. You can install it into an existing page, create a new project or use a third party tool.
There's a pretty thorough installation page in the documentation with a breakdown of the different ways you can install it. The main difference is whether you want to use a tool or install it with a CDN.
The setup syntax is a bit different between the two. There are some good examples you can find that will show you how the code is different.
Create React App
npx create-react-app my-app
When you're building an application, you're going to need some tooling. Tooling includes a developer environment with Live Reloading and other features that make your life easier.
To use this, you'll need to have NodeJS installed, which you can find here.
Once you do that, you can use an npx command to create a new React App. Let's try this out. I'm not using CodeSpaces for this, I'm just using my local computer using a terminal.
Create React App is a nice way to add a development environment and get a decent starter template for your project.
However, it's not what I used for this project. Let's take a look at that.
Vite
- Vite
- HMR
- Sass Support
npm create vite@latest my-react
For this project, I used a new tool called Vite. It's built on top of a bundler like Webpack called Rollup.
It has a lot of newer features like Hot Module Replacement or HMR, which makes it load super fast during development.
It also has built in support for Sass out of the box, which is particularly useful for this project, since PicoCSS can be customized with Sass.
You install it with an npm command, so you need nodejs as well, but it's a little gentler on the install and gives you more options.
Customizing Styles
So far, we've been using inline styles for customizing the css. Let's talk about how you can customize your React projects with regular CSS.
CSS Files
Although I've been using inline styles. Sometimes they don't make sense when using React. It makes more sense to use a separate CSS file when reusing styles or trying to affect entire tags.
For example, I wouldn't mind the headlines having a different color that's sort of a highlight.
We can do that with simple CSS.
h1,
h2,
h3,
h4,
h5,
h6,
b,
summary,
a {
color: #d81b60;
}
a {
color: #d81b60;
cursor: pointer;
}
The PicoCSS site also has a great place where you can control some of the basic colors for the site.
It smartly uses CSS variables that allow you to customize the colors of the site.
You can see all of the available variables at this URL
Now, I have forced the page to publish in light mode. It looks a bit better on these demos.
I did that by adding an attribute to the HTML tag in our index.html file.
<html lang="en" data-theme="light">
If you take that off, it will respond to the system settings.
Introduction to APIs
In the course "Introduction to Web APIs" on LinkedIn Learning, I learned about the basics of Web APIs and how they can be used to build modern web applications. The course covered HTTP requests and responses, JSON, and RESTful APIs. I appreciated the instructor's clear explanations and real-world examples, which helped me better understand how APIs work and how to use them in my projects.
The course started with an overview of HTTP requests and responses, which are the foundation of the web. The instructor did a great job of breaking down the different parts of an HTTP request and response and explaining how they work together. I also learned about JSON, a lightweight data format commonly used in APIs. The instructor provided reading and writing JSON data examples, which helped me understand how APIs work.
The course then moved on to RESTful APIs, a popular way to build APIs. The instructor explained the principles of REST and how to design RESTful APIs. I appreciated the course's hands-on approach, as I could follow along with the instructor and practice what I learned as I went.
Overall, "Introduction to Web APIs" is an excellent course for anyone learning about APIs and how to use them in modern web development. The instructor's clear explanations and practical examples make it easy to follow along, even for beginners. By the end of the course, I felt confident in my ability to build and use APIs in my projects, and I highly recommend this course to anyone looking to do the same.
LINKEDIN TECH TRENDS
According to the LinkedIn Learning Tech Trends course, generative AI is a technology that enables machines to generate new ideas, designs, and even stories through algorithms. This technology is becoming increasingly popular, allowing businesses and individuals to automate tasks and create new content without human intervention. However, there are also concerns about the ethical implications of generative AI, particularly regarding copyright and ownership of generated content. Despite these concerns, many experts predict that generative AI will continue to be a significant trend in the tech industry in the coming years. What stood out to me the most was hearing the phrase referenced repeatedly. That phrase was you wouldn't be replaced by AI but rather by someone who knows and uses AI effectively.
Zero To Mastery Ethical Hacking Course
What is ethical Hacking?
Ethical hacking, also known as "white hat" hacking or penetration testing, is the practice of using the same methods and techniques as malicious hackers, but in a lawful and legitimate manner. It is done in order to find and resolve computer security vulnerabilities and strengthen the security of an organization's networks and systems. In ethical hacking, the hacker does not intend to gain unauthorized access to systems or data, but instead focuses on identifying gaps in security and establishing safeguards against malicious attacks.
What is a virtual Machine?
A virtual machine is a software environment that emulates a physical machine, allowing you to run multiple operating systems or instances of the same operating system on a single physical machine. This can be useful for testing software, running legacy applications, or isolating different environments from one another.
Why Linux?
Linux is the operating system of choice for ethical hacking for a few reasons. Firstly, Linux is open source, which means that anyone can access and modify the source code. This makes it easier for ethical hackers to identify and patch vulnerabilities. Additionally, Linux is highly customizable, making it easier to configure for specific tasks. Finally, many of the tools and scripts used in ethical hacking are designed to run on Linux, making it a natural choice for those working in this field.
How do we create a virtual machine?
To create a virtual machine, you can use software like VirtualBox. First, download and install VirtualBox on your computer. Then, open the software and click on "New" to create a new virtual machine. Follow the prompts to select the operating system you want to install, allocate system resources like RAM and storage, and create a virtual hard disk. Once you have created your virtual machine, you can install the operating system and any necessary software just as you would on a physical machine.
To create a virtual machine with VMware Fusion, follow these steps:
- Open VMware Fusion and click on "New" to create a new virtual machine.
- Select the type of virtual machine you want to create, such as "Windows" or "Linux."
- Choose the installation method you want to use, such as installing from a CD or DVD or using an ISO file.
- Follow the prompts to set up your virtual machine, including selecting the operating system you want to install, allocating system resources like RAM and storage, and creating a virtual hard disk.
- Once you have created your virtual machine, you can install the operating system and any necessary software just as you would on a physical machine.
Kali Linux and VMware Fusion
Kali Linux is a popular Linux distribution for ethical hacking and penetration testing. It comes preloaded with a variety of tools and scripts that are commonly used in this field. To use Kali Linux in a virtual machine, you can use software like VMware Fusion.
To set up Kali Linux in VMware Fusion, follow these steps:
- Download the Kali Linux ISO file from the official website.
- Open VMware Fusion and click on "New" to create a new virtual machine.
- Select "Install from disc or image" and choose the Kali Linux ISO file as the installation source.
- Follow the prompts to set up your virtual machine, including selecting the operating system you want to install, allocating system resources like RAM and storage, and creating a virtual hard disk.
- Once you have created your virtual machine, you can install Kali Linux and any necessary software just as you would on a physical machine.
Using Kali Linux in a virtual machine allows you to experiment with different tools and techniques in a safe and controlled environment. It also allows you to easily create and restore snapshots of your virtual machine, so you can quickly revert back to a previous state if something goes wrong.
Documenting Module 2 Concepts
Link to my notes on Notion for key concepts we are learning in Module 2
Activities this week and Week 10 coming up
The weekend was filled with resting with my family and hanging out with Alaskan friends who have been living in Santa Rosa de Cabal for several years. We had the opportunity to visit them at their house in the country and enjoy a great barbecue hosted by them. We even found an excellent lot for sale, and I hope this could be a potential place to buy and build upon in the near future. At the event, we explored a little nightlife in Pereira, Colombia, and danced for 4 hours in a discoteca all music Colombia with the 90s, reggaeton, bachata, salsa, and of course, Shakira.
I'm looking forward to getting back into the routine of the Hack Reactor program and continually learning and utilizing my React skills, and generating positive momentum for our Module 2 exam.
Ready to work with Xander Clemens?
I'd be happy to discuss your project and how we can work together to create unique, fun and engaging content.
Go ahead and click here to be taken to my business service page and see what I can do for you. Book a call with me now. Looking forward to chatting soon!
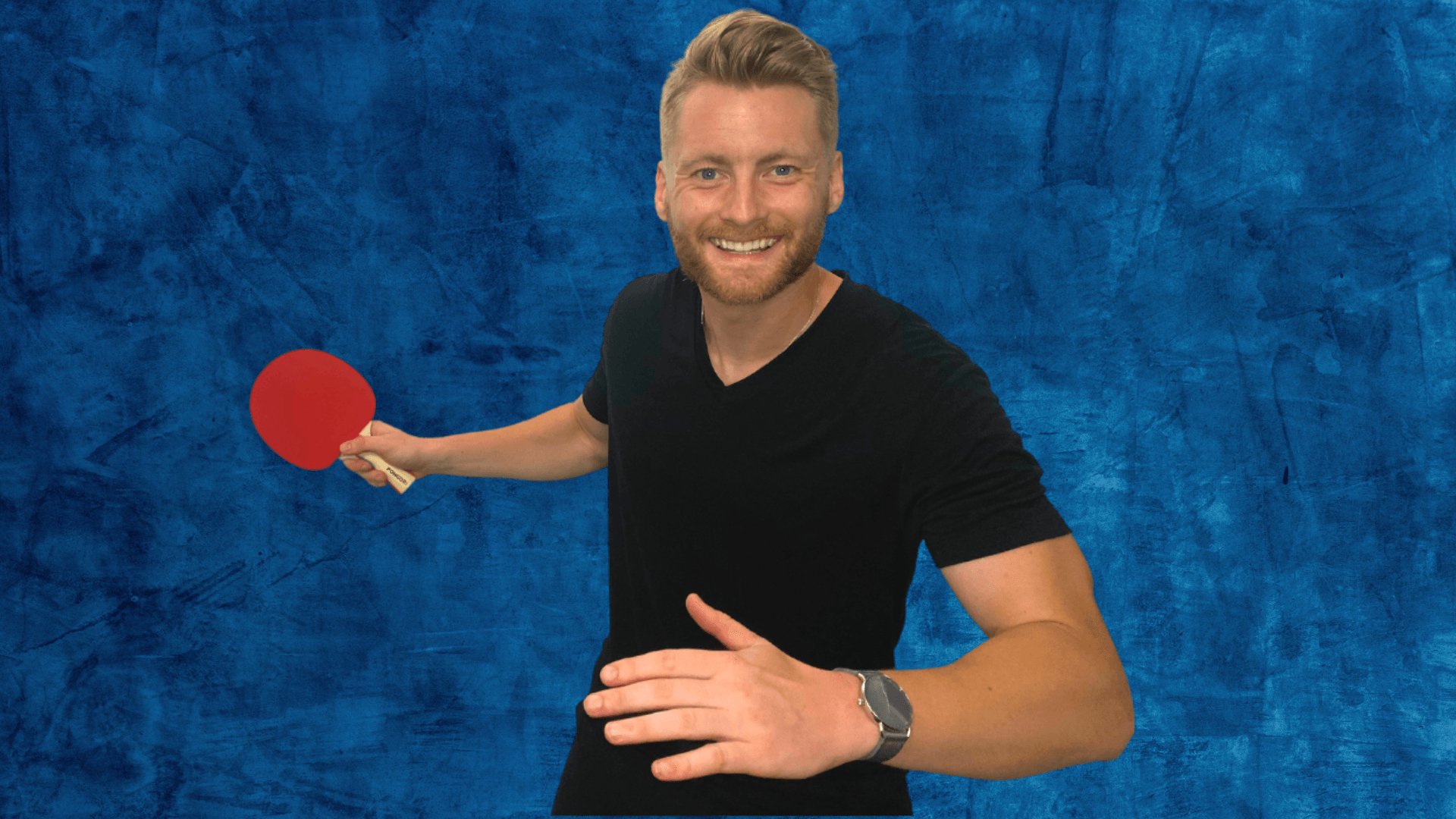